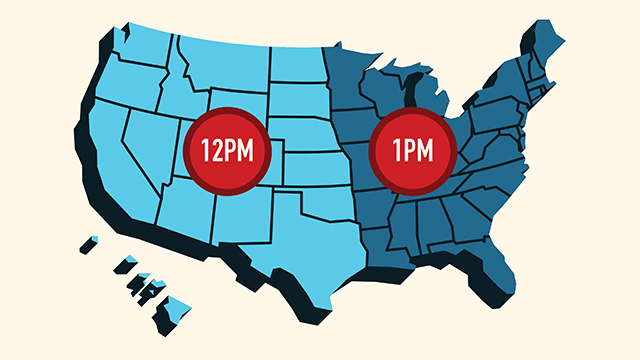
Imagine a scenario where an employee is accused of arriving late to work, but they swear they arrived on time. Upon investigating the audit logs, you notice that the timestamp shows them arriving an hour later than they claimed. However, after further investigation, you realize that the timestamp was incorrectly adjusted for British Summer Time (BST), resulting in an inaccurate record. The employee was actually on time, but the incorrect timestamp led to a false accusation. This scenario highlights the importance of accurately handling timestamps, especially when dealing with daylight saving time (DST) adjustments. Inaccurate timestamps can lead to serious audit problems, wrong accusations, and even legal issues. In this tutorial, we’ll explore how to handle timestamps in Laravel, including accounting for BST and storing timestamps in UTC to prevent such errors and ensure a reliable audit trail.
Accounting for BST
To account for BST, we can use the `Carbon` library, which is included in Laravel. Here’s an example code snippet:
use Carbon\Carbon;
// Get the current date and time
$date = Carbon::now();
// Check if the date falls within the BST period
if ($date->isBetween(Carbon::parse('last Sunday in March'), Carbon::parse('last Sunday in October')))
{
// Add one hour to the date
$date->addHour();
}
// Save the date to the database
YourModel::create(['date' => $date]);
This code checks if the current date falls within the BST period and adds one hour if it does.
Storing Timestamps in UTC
For auditing purposes, it’s generally better to store timestamps in UTC rather than a specific regional timezone like Europe/London. Here’s why:
– Consistency: UTC is a universal standard that doesn’t observe DST or other seasonal adjustments.
– Unambiguous: UTC timestamps are unambiguous and easy to interpret.
– Easier comparison: With UTC timestamps, it’s simpler to compare and correlate events across different systems or locations.
– Audit trail integrity: Using UTC ensures that the audit trail remains intact and tamper-proof.
To store timestamps in UTC, simply set the timezone in your `app.php` config file to `UTC`:
'timezone' => 'UTC',
This will ensure that all timestamps are stored in UTC, regardless of the user’s location or timezone.
Conclusion
In this article, we’ve seen how to handle timestamps in Laravel, including accounting for BST (or any other timezone) and storing timestamps in UTC for auditing purposes. By following these best practices, you can ensure that your timestamps are consistent, reliable, and tamper-proof, preventing errors and wrong accusations. Remember, accurate timestamps are crucial in auditing and logging, so make sure to handle them correctly.
Leave a Reply